Running in both 64 bit and 32 bit Architectures
If you have an assembly built with the Any CPU target platform in Visual Studio then when the application runs in 64 bit process it would run as a 64 bit image like wise if it runs in a 32 bit process it runs as a 32 bit image.
Loading assemblies
If you have an assembly build as x86 target platform a 64 bit process can still load this assembly but the contrary is not true if you have built an assembly in x64 platform this assembly can't be loaded in a 32 bit process
Having both 64 bit and 32 bit build target platform
If you have some projects that target 32 bit platform and other projects that target 64 bit in Visual studio, if you have your target platform as Any CPU, the projects with x86 would not be built and would be skipped.
The same holds for any specific targets for projects within a solution.
In order to build Any CPU, x64 and x86 target platforms in one go you would need to create a new configuration for mixed mode and change individual projects to their required CPU architectures.
If you have an assembly and would like to find out which target platform it was built then use the corflags.exe tool that comes with .NET Framework SDK
Wednesday, July 28, 2010
Tuesday, July 27, 2010
Check if anybody is listening on a port
When we have remoting clients or windows servies we would like to know which ports are free or which programs are using or listening on a port.
We could make use of a program called TCP View from Sysinternal to see all the processes and the ports that they listen to.
Another built in way is to use the windows netstat
In order to search if there are any ports that are listening we could use
netstat -an |find "9099"
Here we are checking if anyone is listening on port 9099 if we did not get any result the ports may not be active but still registered so we are not guaranteed that these ports are free.
We could make use of a program called TCP View from Sysinternal to see all the processes and the ports that they listen to.
Another built in way is to use the windows netstat
In order to search if there are any ports that are listening we could use
netstat -an |find "9099"
Here we are checking if anyone is listening on port 9099 if we did not get any result the ports may not be active but still registered so we are not guaranteed that these ports are free.
Friday, July 23, 2010
Windows 2003 Firewall Changes
Expose Web Site to the Internet, Intranet
Since the web site is exposed on port 80 we need to add this as an exception to the firewall.
Start->Run->Firewall.cpl
Under the exception tab
Click Add Port
Enter Name as "Internet"
Port Number as 80
Make sure its a TCP port.

Check this exception on the list
UNC Path
If we want to share files across UNC Path (\\ServerName\C$\) the firewall should have an exceptions to allow File and Printer Sharing.
Start->Run->Firewall.cpl
Under the exception tab check the File and Printer Sharing.
Since the web site is exposed on port 80 we need to add this as an exception to the firewall.
Start->Run->Firewall.cpl
Under the exception tab
Click Add Port
Enter Name as "Internet"
Port Number as 80
Make sure its a TCP port.

Check this exception on the list
UNC Path
If we want to share files across UNC Path (\\ServerName\C$\) the firewall should have an exceptions to allow File and Printer Sharing.
Start->Run->Firewall.cpl
Under the exception tab check the File and Printer Sharing.

Wednesday, July 21, 2010
Windbg Command Commands
QuickStart
Set up symbol path
File -> Symbol File Path
give the value
srv*C:\MicrosoftSourceCode\RefSrc\Symbols*http://msdl.microsoft.com/download/symbols
where C:\MicrosoftSourceCode\RefSrc\Symbols is the location where the debug symbols would be saved
Attach to the process from File >Attach to a process
To break into a process
Press Key Ctrl+Break
To make the process run
press key g
Switch back and forth between these to debug and run.
To create a User Dump Complete
.dump /ma pathtodumpfile
eg: .dump /ma C:\Software\Findings\App21July2010.dmp
To load SOS based on Microsoft framework
.loadby sos mscorwks
To load the extension on SOS (Son Of Strike) manual
eg: .load C:\Software\SOSExtentions\sosex_32\sosex.dll
To get a list of app domains created
!dumpdomain stat
To get a list of all modules (dlls) loaded
!dumpdomain
get the module address from here and do a
!dumpmodule address
to get what is in the contents do
get the meta data of the assembly
dc <MetaDataStart> <MetaDataEnd>
eg: dc 114d09e4 114d09e4+0n4184
Get the no of GC or Loader heaps in the process
Sos2 Dlls (sos2 is an extension to sos.dll
.load C:\Software\WinDebugger\sos2\sos2.dll
Get a list of module loaded and their sizes
!list -t ntdll!_LIST_ENTRY.Flink -x "dt ntdll!_LDR_DATA_TABLE_ENTRY @$extret\" 005d40b8
Heap Statistics
!dumpheap -stat
Set up symbol path
File -> Symbol File Path
give the value
srv*C:\MicrosoftSourceCode\RefSrc\Symbols*http://msdl.microsoft.com/download/symbols
where C:\MicrosoftSourceCode\RefSrc\Symbols is the location where the debug symbols would be saved
Attach to the process from File >Attach to a process
To break into a process
Press Key Ctrl+Break
To make the process run
press key g
Switch back and forth between these to debug and run.
To create a User Dump Complete
.dump /ma pathtodumpfile
eg: .dump /ma C:\Software\Findings\App21July2010.dmp
To load SOS based on Microsoft framework
.loadby sos mscorwks
To load the extension on SOS (Son Of Strike) manual
eg: .load C:\Software\SOSExtentions\sosex_32\sosex.dll
To get a list of app domains created
!dumpdomain stat
To get a list of all modules (dlls) loaded
!dumpdomain
get the module address from here and do a
!dumpmodule address
to get what is in the contents do
get the meta data of the assembly
dc <MetaDataStart> <MetaDataEnd>
eg: dc 114d09e4 114d09e4+0n4184
Get the no of GC or Loader heaps in the process
Sos2 Dlls (sos2 is an extension to sos.dll
.load C:\Software\WinDebugger\sos2\sos2.dll
Get a list of module loaded and their sizes
!list -t ntdll!_LIST_ENTRY.Flink -x "dt ntdll!_LDR_DATA_TABLE_ENTRY @$extret\" 005d40b8
Heap Statistics
!dumpheap -stat
WinDbg SOS Find Strings in .NET Application
Find all strings loaded in the .net process
!dumpheap -strings
Output for dumping all strings, the total size is the parameter for min and max
Find all the string between specific sizes with and ----- between each listings
.foreach(loadedString {!dumpheap -type System.String -min 40 -max 50 -short}){!do -nofields loadedString;.echo -------------}
Make sure that the range is small as if its too large the windows debugger hangs.
Its better to use the previous command and find out the length of the string and then use the
!dumpheap -type System.String -min 40 -max 50 -short to get the address of these strings
Output for this would be
Name: System.String
MethodTable: 703588c0
EEClass: 7011a498
Size: 44(0x2c) bytes
(C:\Windows\assembly\GAC_32\mscorlib\2.0.0.0__b77a5c561934e089\mscorlib.dll)
String: eventMappings
----------------
since we have put -nofields we don't see the internal fields for String class. The "eventMappings" is the actual value present in the memory
P.S
The blanks are due to the editor not able to understand the table tag
!dumpheap -strings
Output for dumping all strings, the total size is the parameter for min and max
Count | Total Size | String Value |
1 | 20 | "z" |
1 | 20 | "y" |
Find all the string between specific sizes with and ----- between each listings
.foreach(loadedString {!dumpheap -type System.String -min 40 -max 50 -short}){!do -nofields loadedString;.echo -------------}
Make sure that the range is small as if its too large the windows debugger hangs.
Its better to use the previous command and find out the length of the string and then use the
!dumpheap -type System.String -min 40 -max 50 -short to get the address of these strings
Output for this would be
Name: System.String
MethodTable: 703588c0
EEClass: 7011a498
Size: 44(0x2c) bytes
(C:\Windows\assembly\GAC_32\mscorlib\2.0.0.0__b77a5c561934e089\mscorlib.dll)
String: eventMappings
----------------
since we have put -nofields we don't see the internal fields for String class. The "eventMappings" is the actual value present in the memory
P.S
The blanks are due to the editor not able to understand the table tag
Monday, July 19, 2010
Reading SQL Server Roll Over Trace Files into Table
The default roll over files for SQL Server 2005 Trace do not get imported by the fn_trace_gettable if this roll over is done through the trace UI. To fix this problem do the following.
Create a table to hold the trace table
SELECT * INTO Trace071910
FROM fn_trace_gettable('E:\trace_071910\Monitoring_071910.trc', default);
GO
To insert the first trace file records in to the table
Then insert the other records starting from the roll over file which starts with _1, this would start inserting all the records till the end of the trace roll over file is reached.
INSERT INTO Trace071910
SELECT *
FROM fn_trace_gettable('E:\trace_071910\Monitoring_071910_1.trc', default);
GO
In the event of the duration column not available on the trace file we can update it into the trace table with the following query
UPDATE Trace071910
SET duration = DATEDIFF (ms ,startTime ,endTime)
Here I have converted the duration to a millisecond.
Create a table to hold the trace table
SELECT * INTO Trace071910
FROM fn_trace_gettable('E:\trace_071910\Monitoring_071910.trc', default);
GO
To insert the first trace file records in to the table
Then insert the other records starting from the roll over file which starts with _1, this would start inserting all the records till the end of the trace roll over file is reached.
INSERT INTO Trace071910
SELECT *
FROM fn_trace_gettable('E:\trace_071910\Monitoring_071910_1.trc', default);
GO
In the event of the duration column not available on the trace file we can update it into the trace table with the following query
UPDATE Trace071910
SET duration = DATEDIFF (ms ,startTime ,endTime)
Here I have converted the duration to a millisecond.
Sunday, July 18, 2010
Powershell Hyper-V Commands
Check the version of Powershell in Hyper-V.
Type $Host.version
It should be version 2.0
Install the Hyper-V Module Scripts from PSHyperV_Install.zip from CodePlex
UnZip and copy the underlying HyperV_Install folder contents to the Documents/windowsPowershell/HyperV folder of the administrator, the full path should look like
c:\Users\Administrator\Documents\windowsPowershell\modules\HyperV
You could do the same to other users as well or use the installer provided to load to all users with a manual setup.
On the Hyper-V Command Prompt type Powershell to go to the powershell prompt
Type
PS>Import-Module HyperV to load the Hyper V module commands
PS>Get-VMSummary to see a list of Virtual Machines on the box
PS>Start-VM "VMElementName" to start the Machine if its not running.
Type $Host.version
It should be version 2.0
Install the Hyper-V Module Scripts from PSHyperV_Install.zip from CodePlex
UnZip and copy the underlying HyperV_Install folder contents to the Documents/windowsPowershell/HyperV folder of the administrator, the full path should look like
c:\Users\Administrator\Documents\windowsPowershell\modules\HyperV
You could do the same to other users as well or use the installer provided to load to all users with a manual setup.
On the Hyper-V Command Prompt type Powershell to go to the powershell prompt
Type
PS>Import-Module HyperV to load the Hyper V module commands
PS>Get-VMSummary to see a list of Virtual Machines on the box
PS>Start-VM "VMElementName" to start the Machine if its not running.
Thursday, July 15, 2010
Read Only User for SQL Server
In order to create a Readonly user to have just Read out of the CRUD operations for DML Statements
1. Open Security->Logins
2. Right Click the User and choose Properties
3. Click User Mapping
4. On the Database list select the database the user is going to get associated with (Note in SQL Server 2005 the title of this panel is wrongly given as "Users Mapped to this login" instead of "Databases Mapped to this login"
5. On the bottom select the following permissions
1. Open Security->Logins
2. Right Click the User and choose Properties
3. Click User Mapping
4. On the Database list select the database the user is going to get associated with (Note in SQL Server 2005 the title of this panel is wrongly given as "Users Mapped to this login" instead of "Databases Mapped to this login"
5. On the bottom select the following permissions
- db_datareader
- db_denydatawriter
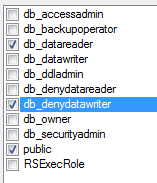
Wednesday, July 14, 2010
OleDbConnection String for Excel, CSV and Tab Delimited Formats
Office 2003 and Lower till 97
Excel Format (xls)
Provider=Microsoft.Jet.OLEDB.4.0;Data Source=FilePathWithFileName;Extended Properties='Excel 8.0;HDR=YES;IMEX=1'
csv Format
Provider=Microsoft.Jet.OLEDB.4.0;Data Source=FilePathWithoutFileName;Extended Properties='text;HDR=YES;FMT=CSVDelimited'
Tab Delimited
Provider=Microsoft.Jet.OLEDB.4.0;Data Source=FilePathWithoutFileName;Extended Properties='text;HDR=YES;FMT=TabDelimited'
Office 2007 and 2010
Excel Format (xlsx)
Provider=Microsoft.ACE.OLEDB.12.0;Data Source=FilePathWithFileName;Extended Properties=""Excel 12.0;HDR=Yes;IMEX=2
csv Format
Provider=Microsoft.ACE.OLEDB.12.0;Data Source=FilePathWithoutFileName;Extended Properties='text;HDR=YES;FMT=CSVDelimited'
Tab Delimited
Provider=Microsoft.ACE.OLEDB.12.0;Data Source=FilePathWithoutFileName;Extended Properties='text;HDR=YES;FMT=TabDelimited'
Note For Office 2010 you would need to download
Microsoft Access Database Engine 2010
If this is installed this is backward compatible to read older versions too (xls) so there is no need for having two different connection string.
Since this driver has a 64 bit version, the long standing bottleneck of not able to use the 64 bit CPU architecture is finally solved.
Excel Format (xls)
Provider=Microsoft.Jet.OLEDB.4.0;Data Source=FilePathWithFileName;Extended Properties='Excel 8.0;HDR=YES;IMEX=1'
csv Format
Provider=Microsoft.Jet.OLEDB.4.0;Data Source=FilePathWithoutFileName;Extended Properties='text;HDR=YES;FMT=CSVDelimited'
Tab Delimited
Provider=Microsoft.Jet.OLEDB.4.0;Data Source=FilePathWithoutFileName;Extended Properties='text;HDR=YES;FMT=TabDelimited'
Office 2007 and 2010
Excel Format (xlsx)
Provider=Microsoft.ACE.OLEDB.12.0;Data Source=FilePathWithFileName;Extended Properties=""Excel 12.0;HDR=Yes;IMEX=2
csv Format
Provider=Microsoft.ACE.OLEDB.12.0;Data Source=FilePathWithoutFileName;Extended Properties='text;HDR=YES;FMT=CSVDelimited'
Tab Delimited
Provider=Microsoft.ACE.OLEDB.12.0;Data Source=FilePathWithoutFileName;Extended Properties='text;HDR=YES;FMT=TabDelimited'
Note For Office 2010 you would need to download
Microsoft Access Database Engine 2010
If this is installed this is backward compatible to read older versions too (xls) so there is no need for having two different connection string.
Since this driver has a 64 bit version, the long standing bottleneck of not able to use the 64 bit CPU architecture is finally solved.
Gotomeeting Outlook Plugin
This plugin is not a stand alone install.
This needs to be installed as part of the entire application.
Navigate to
www.gotomeeting.com
click on "Host a meeting" at the top of the page
This would give a page with a button which says "Launch Software"
This would install the software together with the Outlook toolbar. If you had Outlook opened while the installation was on, you have to restart Outlook to see the tool bar.
This needs to be installed as part of the entire application.
Navigate to
www.gotomeeting.com
click on "Host a meeting" at the top of the page
This would give a page with a button which says "Launch Software"
This would install the software together with the Outlook toolbar. If you had Outlook opened while the installation was on, you have to restart Outlook to see the tool bar.

Monday, July 12, 2010
Hyper-V and Diskpart to extend an existing virtual hard disk
Using Hyper-V make sure that the virtual disk is not partitioned. If you want a new partition instead of partitioning the disk create a new disk and add it to the virtual machine.
If however the existing disk space is not enough we may need to increase the disk size using Hyper V Manager
1. Open Hyper V Manager
2. On the list of Virtual Machine make sure the machine whose disk is to be extended is shut down
3. Right click the machine and choose settings
4. On the settings dialog chose the Hard Drive (IDE Controller)
5. Under the Virtual hard disk click on the edit button
6. Under Choose action select expand
7. Under the Configure Disk change the new size to the desired value and save the changes
8. Using the Hyper V Manager Start the virtual machine.
9. If the virtual disk is a partition the newly allocated size would come up as unallocated space in Disk Management
10. We can add this to last partition created on the disk using diskpart
11. Open a command prompt (hopefully with administrative access)
12. Type Diskpart
13. Type list volume
14. Select the volume nearest to the unallocated space in the Disk Management utility discussed previously by giving the command select volume
15. Type extend
The partition would have absorbed the newly unallocated space.
Happy Extending
Anton
If however the existing disk space is not enough we may need to increase the disk size using Hyper V Manager
1. Open Hyper V Manager
2. On the list of Virtual Machine make sure the machine whose disk is to be extended is shut down
3. Right click the machine and choose settings
4. On the settings dialog chose the Hard Drive (IDE Controller)
5. Under the Virtual hard disk click on the edit button
6. Under Choose action select expand
7. Under the Configure Disk change the new size to the desired value and save the changes
8. Using the Hyper V Manager Start the virtual machine.
9. If the virtual disk is a partition the newly allocated size would come up as unallocated space in Disk Management
10. We can add this to last partition created on the disk using diskpart
11. Open a command prompt (hopefully with administrative access)
12. Type Diskpart
13. Type list volume
14. Select the volume nearest to the unallocated space in the Disk Management utility discussed previously by giving the command select volume
15. Type extend
The partition would have absorbed the newly unallocated space.
Happy Extending
Anton
Subscribe to:
Posts (Atom)